NAME
LibUI - Simple, Portable, Native GUI Library
SYNOPSIS
use LibUI ':all';
use LibUI::Window;
use LibUI::Label;
Init( ) && die;
my $window = LibUI::Window->new( 'Hi', 320, 100, 0 );
$window->setMargined( 1 );
$window->setChild( LibUI::Label->new('Hello, World!') );
$window->onClosing(
sub {
Quit();
return 1;
},
undef
);
$window->show;
Main();
Screenshots
Linux
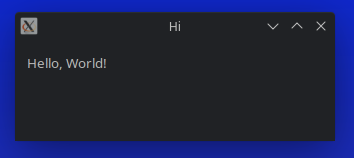
MacOS
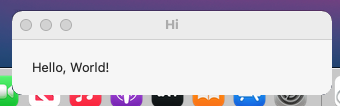
Windows
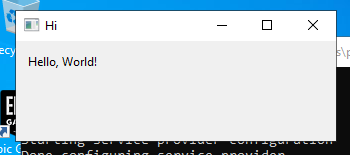
DESCRIPTION
LibUI is a simple and portable (but not inflexible) GUI library in C that uses the native GUI technologies of each platform it supports.
This distribution is under construction. It works but is incomplete.
Container controls
- LibUI::Window - a top-level window
- LibUI::HBox - a horizontally aligned, boxlike container that holds a group of controls
- LibUI::VBox - a vertically aligned, boxlike container that holds a group of controls
- LibUI::Tab - a multi-page control interface that displays one page at a time
- LibUI::Group - a container that adds a label to the child
- LibUI::Form - a container to organize controls as labeled fields
- LibUI::Grid - a container to arrange controls in a grid
Data entry controls
- LibUI::Checkbox - a user checkable box accompanied by a text label
- LibUI::Entry - a single line text entry field
- LibUI::PasswordEntry - a single line, obscured text entry field
- LibUI::SearchEntry - a single line search query field
- LibUI::Slider - display and modify integer values via a draggable slider
- LibUI::DateTimePicker - a control to enter a date and/or time
- LibUI::DatePicker - a control to enter a date
- LibUI::TimePicker - a control to enter a time
- LibUI::MultilineEntry - a multi line entry that visually wraps text when lines overflow
- LibUI::NonWrappingMultilineEntry - a multi line entry that scrolls text horizontally when lines overflow
- LibUI::FontButton - a control that opens a font picker when clicked
Static controls
- LibUI::Label - a control to display non-interactive text
- LibUI::ProgressBar - a control that visualizes the progress of a task via the fill level of a horizontal bar
- LibUI::HSeparator - a control to visually separate controls horizontally
- LibUI::VSeparator - a control to visually separate controls vertically
Dialog windows
openFile( )
- File chooser to select a single fileopenFolder( )
- File chooser to select a single foldersaveFile( )
- Save file dialogmsgBox( ... )
- Message box dialogmsgBoxError( ... )
- Error message box dialog
See "Dialog windows" in LibUI::Window for more.
Menus
Tables
The upstream API is a mess so I'm still plotting around this.
GUI Functions
Some basics you gotta use just to keep a modern GUI running.
This is incomplete but... well, I'm working on it.
Init( [...] )
Init( );
Ask LibUI to do all the platform specific work to get up and running. If LibUI fails to initialize itself, this will return a true value. Weird upstream choice, I know...
You must call this before creating widgets.
Main( ... )
Main( );
Let LibUI's event loop run until interrupted.
Uninit( ... )
Uninit( );
Ask LibUI to break everything down before quitting.
Quit( ... )
Quit( );
Quit.
Timer( ... )
Timer( 1000, sub { die 'do not do this here' }, undef);
Timer(
1000,
sub {
my $data = shift;
return 1 unless ++$data->{ticks} == 5;
0;
},
{ ticks => 0 }
);
Expected parameters include:
$time
-
Time in milliseconds.
$func
-
CodeRef that will be triggered when
$time
runs out.Return a true value from your
$func
to make your timer repeating. $data
-
Any userdata you feel like passing. It'll be handed off to your function.
Requirements
See Alien::libui
See Also
eg/demo.pl - Very basic example
eg/widgets.pl - Demo of basic controls
LICENSE
Copyright (C) Sanko Robinson.
This library is free software; you can redistribute it and/or modify it under the same terms as Perl itself.
AUTHOR
Sanko Robinson <sanko@cpan.org>